It is good for speed in development, 'pretty' URLs, and flexibility when handling data requests and mutations.
This makes for applications that are easily scalable, usable, and independent of other services.
For example, it is obvious that this API request updates the city "Cairns" with the temperature of 27°C:
https://api.weather.com/update_city/Cairns/?temperature=27
Stateless communication means that each request must contain all of the information necessary to be understood by the server, as the server isn't storing any session information about the client.
If I (the client) request Tuesdays roster from my work host KFC (server), I can't then send a request for the 'next' days roster, because the KFC REST server isn't tracking what day it delivered me in my initial request - so the server can't know what 'next' is...
this fails stateless communicationThe server could deliver me both Tuesday's roster as well as explicit URI's to access the resource containing Wednesday's roster (one day after).
this would conform with the REST constraint of stateless communicationAdditionally, if i was a manager, the server may also deliver me a representation of all people working Tuesday, with enough URI's for me to edit or delete (i.e. manipulate) these shifts.
sessions
used to authenticate a client violate the stateless constraint of REST.There are many ways around this (read this), with the basic premise that each new client request also supplies the required credentials, such as an authentication token or a public key.
Token: usually generated on a per-session basis, requiring users to login and authenticate to gain access to a server-generated token (for an example, see OAuth 2.0 Simplified).
Key: authorises usage of a resource for an application, but does not (necessarily) authenticate a user, for example:
https://maps.googleapis.com/maps/api/geocode/json?address=Brisbane&key=AIjlSyEI1n03b7-this-is-fake-5tHcDxy1RnI
Either token or key could be used for secure, stateless requests.
SOAP vs REST APIs
Q: Why use REST design and not SOAP protocol to make an API?
A: JSON via HTTP responses are cacheable, which suits all Digital Solution units that sit within a web context. (truthfully its just because your teacher prefers JSON over XML, because JSON doesn't use an end tag, and is therefore shorter and quicker to read and write, plus JSON can use arrays / lists unlike XML)
RESTful services vs AJAX
- RESTful services
- API URI delivering JSON or XML
- AJAX
- Asynchronous JavaScript and XML
- Uniform Interface, e.g.:
API/rosters/Tuesday/remove/?shift=Night&employee=Barry
- Stateless
- Cacheable (responses can be saved by a client - but for how long? performance vs recency)
- Client-Server (obviously)
- Layered System (so if you are delivering markup or other web services, keep these separate)
Code on demand is optional (so not a requirement of REST) as most of the time servers will send back JSON or XML, but sometimes they may send executable code (we won't be in this course so don't worry about this).
XML
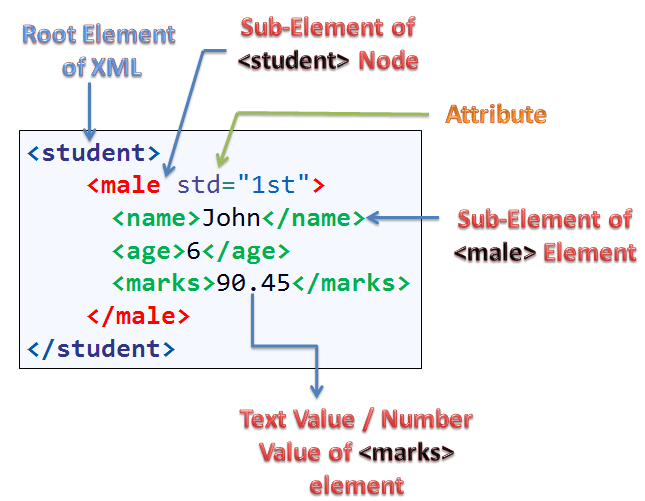
JSON
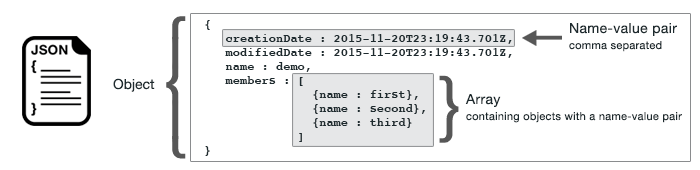